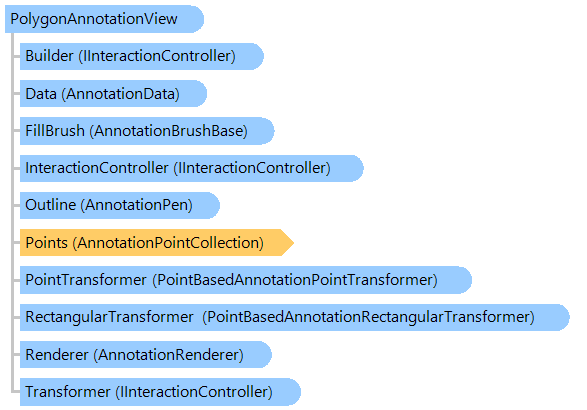
'Declaration Public Class PolygonAnnotationView Inherits LinesAnnotationView
public class PolygonAnnotationView : LinesAnnotationView
public __gc class PolygonAnnotationView : public LinesAnnotationView*
public ref class PolygonAnnotationView : public LinesAnnotationView^
Вот пример, показывающий, как создать пользовательскую многоугольную аннотацию, которая рисует дополнительный текст в аннотации:
#Region "TextPolygonAnnotationData" ''' <summary> ''' Contains information about the annotation that displays polygon with text. ''' </summary> ''' <remarks> ''' This class shows how to create annotation data and annotation view with custom property. ''' </remarks> Public Class TextPolygonAnnotationData Inherits Vintasoft.Imaging.Annotation.PolygonAnnotationData #Region "Constructors" ''' <summary> ''' Initializes a new instance of the <see cref="TextPolygonAnnotationData"/> class. ''' </summary> Public Sub New() MyBase.New() Text = "" Font = New Vintasoft.Imaging.Annotation.AnnotationFont("Arial", 20F) FontBrush = New Vintasoft.Imaging.Annotation.AnnotationSolidBrush(System.Drawing.Color.Black) End Sub ''' <summary> ''' Initializes a new instance of the <see cref="TextPolygonAnnotationData"/> class. ''' </summary> ''' <param name="info">The SerializationInfo to populate with data.</param> ''' <param name="context">The destination for this serialization.</param> Public Sub New(info As System.Runtime.Serialization.SerializationInfo, context As System.Runtime.Serialization.StreamingContext) MyBase.New(info, context) Text = DirectCast(info.GetValue("Text", GetType(String)), String) Font = DirectCast(info.GetValue("Font", GetType(Vintasoft.Imaging.Annotation.AnnotationFont)), Vintasoft.Imaging.Annotation.AnnotationFont) FontBrush = DirectCast(info.GetValue("FontBrush", GetType(Vintasoft.Imaging.Annotation.AnnotationBrushBase)), Vintasoft.Imaging.Annotation.AnnotationBrushBase) End Sub ''' <summary> ''' Initializes the <see cref="TextPolygonAnnotationData"/> class. ''' </summary> Shared Sub New() ' register AnnotationView for AnnotationData Vintasoft.Imaging.Annotation.UI.AnnotationViewFactory.RegisterViewForAnnotationData(GetType(TextPolygonAnnotationData), GetType(TextPolygonAnnotationView)) Vintasoft.Imaging.Annotation.Rendering.AnnotationRendererFactory.RegisterRendererForAnnotationData(GetType(TextPolygonAnnotationData), GetType(TextPolygonAnnotationRenderer)) End Sub #End Region #Region "Properties" Private _text As String ''' <summary> ''' Gets or sets the text of annotation. ''' </summary> <System.ComponentModel.Description("Text of annotation.")> _ Public Property Text() As String Get Return _text End Get Set If _text <> value Then Dim changingArgs As New Vintasoft.Imaging.ObjectPropertyChangingEventArgs("Text", Text, value) ' if action is not canceled If OnPropertyChanging(changingArgs) Then _text = DirectCast(changingArgs.NewValue, String) OnPropertyChanged(changingArgs.ToChangedEventArgs()) End If End If End Set End Property Private _font As Vintasoft.Imaging.Annotation.AnnotationFont ''' <summary> ''' Gets or sets the font of annotation. ''' </summary> <System.ComponentModel.Description("Text font of annotation.")> _ Public Property Font() As Vintasoft.Imaging.Annotation.AnnotationFont Get Return _font End Get Set If value Is Nothing Then Throw New System.ArgumentNullException() End If If _font Is Nothing OrElse Not _font.Equals(value) Then Dim changingArgs As New Vintasoft.Imaging.ObjectPropertyChangingEventArgs("Font", Font, value) ' if action is not canceled If OnPropertyChanging(changingArgs) Then _font = DirectCast(changingArgs.NewValue, Vintasoft.Imaging.Annotation.AnnotationFont) OnPropertyChanged(changingArgs.ToChangedEventArgs()) End If End If End Set End Property Private _fontBrush As Vintasoft.Imaging.Annotation.AnnotationBrushBase ''' <summary> ''' Gets or sets the font brush of annotation. ''' </summary> <System.ComponentModel.Description("Font brush of annotation.")> _ Public Property FontBrush() As Vintasoft.Imaging.Annotation.AnnotationBrushBase Get Return _fontBrush End Get Set If _fontBrush IsNot value Then Dim changingArgs As New Vintasoft.Imaging.ObjectPropertyChangingEventArgs("FontBrush", FontBrush, value) ' if action is not canceled If OnPropertyChanging(changingArgs) Then _fontBrush = DirectCast(changingArgs.NewValue, Vintasoft.Imaging.Annotation.AnnotationBrushBase) OnPropertyChanged(changingArgs.ToChangedEventArgs()) End If End If End Set End Property #End Region #Region "Methods" ''' <summary> ''' Populates a SerializationInfo with the data needed to serialize the target object. ''' </summary> ''' <param name="info">The SerializationInfo to populate with data.</param> ''' <param name="context">The destination for this serialization.</param> Public Overrides Sub GetObjectData(info As System.Runtime.Serialization.SerializationInfo, context As System.Runtime.Serialization.StreamingContext) MyBase.GetObjectData(info, context) info.AddValue("Text", Text) info.AddValue("Font", Font) info.AddValue("FontBrush", FontBrush) End Sub ''' <summary> ''' Copies the state of the current object to the target object. ''' </summary> ''' <param name="target">Object to copy the state of the current object to.</param> Public Overrides Sub CopyTo(target As Vintasoft.Imaging.Annotation.AnnotationData) Dim typedTarget As TextPolygonAnnotationData = DirectCast(target, TextPolygonAnnotationData) MyBase.CopyTo(typedTarget) typedTarget.Text = Text typedTarget.Font = DirectCast(Font.Clone(), Vintasoft.Imaging.Annotation.AnnotationFont) typedTarget.FontBrush = DirectCast(FontBrush.Clone(), Vintasoft.Imaging.Annotation.AnnotationBrushBase) End Sub ''' <summary> ''' Creates a new object that is a copy of the current ''' <see cref="TextPolygonAnnotationData" /> instance. ''' </summary> ''' <returns> ''' A new object that is a copy of this ''' <see cref="TextPolygonAnnotationData" /> instance. ''' </returns> Public Overrides Function Clone() As Object Dim result As New TextPolygonAnnotationData() Me.CopyTo(result) Return result End Function #End Region End Class #End Region #Region "TextPolygonAnnotationRenderer" ''' <summary> ''' Determines how to render the <see cref="TextPolygonAnnotationData"/>. ''' </summary> Public Class TextPolygonAnnotationRenderer Inherits Vintasoft.Imaging.Annotation.Rendering.PolygonAnnotationRenderer #Region "Constructors" ''' <summary> ''' Initializes a new instance of the <see cref="TextPolygonAnnotationRenderer"/>. ''' </summary> ''' <param name="annotationData">Object that stores the annotation data.</param> Public Sub New(annotationData As TextPolygonAnnotationData) MyBase.New(annotationData) End Sub #End Region #Region "Methods" ''' <summary> ''' Renders the annotation on the <see cref="Vintasoft.Imaging.Drawing.DrawingEngine"/> in the ''' coordinate space of annotation. ''' </summary> ''' <param name="drawingEngine">The <see cref="Vintasoft.Imaging.Drawing.DrawingEngine"/> to render on.</param> ''' <param name="drawingSurface">The object that provides information about drawing surface.</param> Protected Overrides Sub RenderInContentSpace(drawingEngine As Vintasoft.Imaging.Drawing.DrawingEngine, drawingSurface As Vintasoft.Imaging.DrawingSurface) MyBase.RenderInContentSpace(drawingEngine, drawingSurface) Dim data__1 As TextPolygonAnnotationData = DirectCast(Data, TextPolygonAnnotationData) If data__1.Font Is Nothing OrElse data__1.FontBrush Is Nothing OrElse data__1.Text Is Nothing OrElse data__1.Text = "" Then Return End If ' create font brush Using fontBrush As Vintasoft.Imaging.Drawing.IDrawingBrush = Vintasoft.Imaging.Drawing.DrawingFactoryAnnotationsExtensions.CreateBrush(drawingEngine.DrawingFactory, data__1.FontBrush) If fontBrush Is Nothing Then Return End If ' annotation size Dim width As Single = data__1.Size.Width Dim height As Single = data__1.Size.Height ' calculate text rectangle Dim textRectangle As System.Drawing.RectangleF = GetBoundingBox(data__1.Points) Using drawingEngine.CreateClipHolder() Using drawingEngine.CreateTransformHolder() ' set clip drawingEngine.CombineClip(textRectangle, Vintasoft.Imaging.Drawing.RegionCombineMode.Intersect) ' if annotation is mirrored horizontally If data__1.HorizontalMirrored Then ' change the world transformation of drawing engine drawingEngine.MultiplyTransformPrepend(Vintasoft.Imaging.AffineMatrix.CreateScaling(-1, 1)) End If ' if annotation is mirrored vertically If data__1.VerticalMirrored Then ' change the world transformation of drawing engine drawingEngine.MultiplyTransformPrepend(Vintasoft.Imaging.AffineMatrix.CreateScaling(1, -1)) End If ' create string format for text Dim layoutProperties As New Vintasoft.Imaging.Drawing.TextLayoutProperties(Vintasoft.Imaging.AnchorType.Center) ' create font for drawing text Using drawingFont As Vintasoft.Imaging.Drawing.IDrawingFont = Vintasoft.Imaging.Drawing.DrawingFactoryAnnotationsExtensions.CreateFont(drawingEngine.DrawingFactory, data__1.Font, layoutProperties) ' draw text drawingEngine.DrawText(data__1.Text, drawingFont, fontBrush, textRectangle, layoutProperties) End Using End Using End Using End Using End Sub ''' <summary> ''' Returns the bounding box of specified polygon. ''' </summary> ''' <param name="points">The polygon points.</param> ''' <returns>Bounding box of polygon.</returns> Private Shared Function GetBoundingBox(points As System.Collections.Generic.IList(Of System.Drawing.PointF)) As System.Drawing.RectangleF If points.Count = 0 Then Return System.Drawing.RectangleF.Empty End If Dim x1 As Single, x2 As Single, y1 As Single, y2 As Single x1 = InlineAssignHelper(x2, points(0).X) y1 = InlineAssignHelper(y2, points(0).Y) For i As Integer = 1 To points.Count - 1 Dim x As Single = points(i).X Dim y As Single = points(i).Y If x1 > x Then x1 = x End If If x2 < x Then x2 = x End If If y1 > y Then y1 = y End If If y2 < y Then y2 = y End If Next Return New System.Drawing.RectangleF(x1, y1, x2 - x1, y2 - y1) End Function Private Shared Function InlineAssignHelper(Of T)(ByRef target As T, value As T) As T target = value Return value End Function #End Region End Class #End Region #Region "TextPolygonAnnotationView" ''' <summary> ''' Determines how to display the annotation that displays polygon with text. ''' </summary> Public Class TextPolygonAnnotationView Inherits Vintasoft.Imaging.Annotation.UI.PolygonAnnotationView #Region "Constructors" ''' <summary> ''' Initializes a new instance of the <see cref="TextPolygonAnnotationView"/> class. ''' </summary> ''' <param name="annotationData">Object that stores the annotation data.</param> Public Sub New(annotationData As TextPolygonAnnotationData) MyBase.New(annotationData) End Sub #End Region #Region "Properties" ''' <summary> ''' Gets or sets the text of the annotation. ''' </summary> <System.ComponentModel.Description("Text of the annotation.")> _ Public Property Text() As String Get Return TextAnnoData.Text End Get Set TextAnnoData.Text = value End Set End Property ''' <summary> ''' Gets or sets the <see cref="Font"/> of the annotation. ''' </summary> <System.ComponentModel.Description("Text font of the annotation.")> _ Public Property Font() As Vintasoft.Imaging.Annotation.AnnotationFont Get Return TextAnnoData.Font End Get Set TextAnnoData.Font = value End Set End Property ''' <summary> ''' Gets or sets the font brush of the annotation. ''' </summary> <System.ComponentModel.Description("Font brush of the annotation.")> _ Public Property FontBrush() As Vintasoft.Imaging.Annotation.AnnotationBrushBase Get Return TextAnnoData.FontBrush End Get Set TextAnnoData.FontBrush = value End Set End Property ''' <summary> ''' Gets typed annotation data value. ''' </summary> Private ReadOnly Property TextAnnoData() As TextPolygonAnnotationData Get Return DirectCast(Data, TextPolygonAnnotationData) End Get End Property #End Region #Region "Methods" ''' <summary> ''' Creates a new object that is a copy of the current ''' <see cref="Vintasoft.Imaging.Annotation.UI.TextAnnotationView"/> instance. ''' </summary> ''' <returns>A new object that is a copy of this ''' <see cref="Vintasoft.Imaging.Annotation.UI.TextAnnotationView"/> instance.</returns> Public Overrides Function Clone() As Object Return New TextPolygonAnnotationView(DirectCast(Data.Clone(), TextPolygonAnnotationData)) End Function #End Region End Class #End Region
#region TextPolygonAnnotationData /// <summary> /// Contains information about the annotation that displays polygon with text. /// </summary> /// <remarks> /// This class shows how to create annotation data and annotation view with custom property. /// </remarks> public class TextPolygonAnnotationData : Vintasoft.Imaging.Annotation.PolygonAnnotationData { #region Constructors /// <summary> /// Initializes a new instance of the <see cref="TextPolygonAnnotationData"/> class. /// </summary> public TextPolygonAnnotationData() : base() { Text = ""; Font = new Vintasoft.Imaging.Annotation.AnnotationFont("Arial", 20f); FontBrush = new Vintasoft.Imaging.Annotation.AnnotationSolidBrush( System.Drawing.Color.Black); } /// <summary> /// Initializes a new instance of the <see cref="TextPolygonAnnotationData"/> class. /// </summary> /// <param name="info">The SerializationInfo to populate with data.</param> /// <param name="context">The destination for this serialization.</param> public TextPolygonAnnotationData(System.Runtime.Serialization.SerializationInfo info, System.Runtime.Serialization.StreamingContext context) : base(info, context) { Text = (string)info.GetValue("Text", typeof(string)); Font = (Vintasoft.Imaging.Annotation.AnnotationFont)info.GetValue("Font", typeof(Vintasoft.Imaging.Annotation.AnnotationFont)); FontBrush = (Vintasoft.Imaging.Annotation.AnnotationBrushBase)info.GetValue("FontBrush", typeof(Vintasoft.Imaging.Annotation.AnnotationBrushBase)); } /// <summary> /// Initializes the <see cref="TextPolygonAnnotationData"/> class. /// </summary> static TextPolygonAnnotationData() { // register AnnotationView for AnnotationData Vintasoft.Imaging.Annotation.UI.AnnotationViewFactory.RegisterViewForAnnotationData( typeof(TextPolygonAnnotationData), typeof(TextPolygonAnnotationView)); Vintasoft.Imaging.Annotation.Rendering.AnnotationRendererFactory.RegisterRendererForAnnotationData( typeof(TextPolygonAnnotationData), typeof(TextPolygonAnnotationRenderer)); } #endregion #region Properties string _text; /// <summary> /// Gets or sets the text of annotation. /// </summary> [System.ComponentModel.Description("Text of annotation.")] public string Text { get { return _text; } set { if (_text != value) { Vintasoft.Imaging.ObjectPropertyChangingEventArgs changingArgs = new Vintasoft.Imaging.ObjectPropertyChangingEventArgs("Text", Text, value); // if action is not canceled if (OnPropertyChanging(changingArgs)) { _text = (string)changingArgs.NewValue; OnPropertyChanged(changingArgs.ToChangedEventArgs()); } } } } Vintasoft.Imaging.Annotation.AnnotationFont _font; /// <summary> /// Gets or sets the font of annotation. /// </summary> [System.ComponentModel.Description("Text font of annotation.")] public Vintasoft.Imaging.Annotation.AnnotationFont Font { get { return _font; } set { if (value == null) throw new System.ArgumentNullException(); if (_font == null || !_font.Equals(value)) { Vintasoft.Imaging.ObjectPropertyChangingEventArgs changingArgs = new Vintasoft.Imaging.ObjectPropertyChangingEventArgs("Font", Font, value); // if action is not canceled if (OnPropertyChanging(changingArgs)) { _font = (Vintasoft.Imaging.Annotation.AnnotationFont)changingArgs.NewValue; OnPropertyChanged(changingArgs.ToChangedEventArgs()); } } } } Vintasoft.Imaging.Annotation.AnnotationBrushBase _fontBrush; /// <summary> /// Gets or sets the font brush of annotation. /// </summary> [System.ComponentModel.Description("Font brush of annotation.")] public Vintasoft.Imaging.Annotation.AnnotationBrushBase FontBrush { get { return _fontBrush; } set { if (_fontBrush != value) { Vintasoft.Imaging.ObjectPropertyChangingEventArgs changingArgs = new Vintasoft.Imaging.ObjectPropertyChangingEventArgs("FontBrush", FontBrush, value); // if action is not canceled if (OnPropertyChanging(changingArgs)) { _fontBrush = (Vintasoft.Imaging.Annotation.AnnotationBrushBase)changingArgs.NewValue; OnPropertyChanged(changingArgs.ToChangedEventArgs()); } } } } #endregion #region Methods /// <summary> /// Populates a SerializationInfo with the data needed to serialize the target object. /// </summary> /// <param name="info">The SerializationInfo to populate with data.</param> /// <param name="context">The destination for this serialization.</param> public override void GetObjectData(System.Runtime.Serialization.SerializationInfo info, System.Runtime.Serialization.StreamingContext context) { base.GetObjectData(info, context); info.AddValue("Text", Text); info.AddValue("Font", Font); info.AddValue("FontBrush", FontBrush); } /// <summary> /// Copies the state of the current object to the target object. /// </summary> /// <param name="target">Object to copy the state of the current object to.</param> public override void CopyTo(Vintasoft.Imaging.Annotation.AnnotationData target) { TextPolygonAnnotationData typedTarget = (TextPolygonAnnotationData)target; base.CopyTo(typedTarget); typedTarget.Text = Text; typedTarget.Font = (Vintasoft.Imaging.Annotation.AnnotationFont)Font.Clone(); typedTarget.FontBrush = (Vintasoft.Imaging.Annotation.AnnotationBrushBase)FontBrush.Clone(); } /// <summary> /// Creates a new object that is a copy of the current /// <see cref="TextPolygonAnnotationData" /> instance. /// </summary> /// <returns> /// A new object that is a copy of this /// <see cref="TextPolygonAnnotationData" /> instance. /// </returns> public override object Clone() { TextPolygonAnnotationData result = new TextPolygonAnnotationData(); this.CopyTo(result); return result; } #endregion } #endregion #region TextPolygonAnnotationRenderer /// <summary> /// Determines how to render the <see cref="TextPolygonAnnotationData"/>. /// </summary> public class TextPolygonAnnotationRenderer : Vintasoft.Imaging.Annotation.Rendering.PolygonAnnotationRenderer { #region Constructors /// <summary> /// Initializes a new instance of the <see cref="TextPolygonAnnotationRenderer"/>. /// </summary> /// <param name="annotationData">Object that stores the annotation data.</param> public TextPolygonAnnotationRenderer(TextPolygonAnnotationData annotationData) : base(annotationData) { } #endregion #region Methods /// <summary> /// Renders the annotation on the <see cref="Vintasoft.Imaging.Drawing.DrawingEngine"/> in the /// coordinate space of annotation. /// </summary> /// <param name="drawingEngine">The <see cref="Vintasoft.Imaging.Drawing.DrawingEngine"/> to render on.</param> /// <param name="drawingSurface">The object that provides information about drawing surface.</param> protected override void RenderInContentSpace(Vintasoft.Imaging.Drawing.DrawingEngine drawingEngine, Vintasoft.Imaging.DrawingSurface drawingSurface) { base.RenderInContentSpace(drawingEngine, drawingSurface); TextPolygonAnnotationData data = (TextPolygonAnnotationData)Data; if (data.Font == null || data.FontBrush == null || data.Text == null || data.Text == "") return; // create font brush using (Vintasoft.Imaging.Drawing.IDrawingBrush fontBrush = Vintasoft.Imaging.Drawing.DrawingFactoryAnnotationsExtensions.CreateBrush(drawingEngine.DrawingFactory, data.FontBrush)) { if (fontBrush == null) return; // annotation size float width = data.Size.Width; float height = data.Size.Height; // calculate text rectangle System.Drawing.RectangleF textRectangle = GetBoundingBox(data.Points); using (drawingEngine.CreateClipHolder()) { using (drawingEngine.CreateTransformHolder()) { // set clip drawingEngine.CombineClip(textRectangle, Vintasoft.Imaging.Drawing.RegionCombineMode.Intersect); // if annotation is mirrored horizontally if (data.HorizontalMirrored) // change the world transformation of drawing engine drawingEngine.MultiplyTransformPrepend(Vintasoft.Imaging.AffineMatrix.CreateScaling(-1, 1)); // if annotation is mirrored vertically if (data.VerticalMirrored) // change the world transformation of drawing engine drawingEngine.MultiplyTransformPrepend(Vintasoft.Imaging.AffineMatrix.CreateScaling(1, -1)); // create string format for text Vintasoft.Imaging.Drawing.TextLayoutProperties layoutProperties = new Vintasoft.Imaging.Drawing.TextLayoutProperties(Vintasoft.Imaging.AnchorType.Center); // create font for drawing text using (Vintasoft.Imaging.Drawing.IDrawingFont drawingFont = Vintasoft.Imaging.Drawing.DrawingFactoryAnnotationsExtensions.CreateFont(drawingEngine.DrawingFactory, data.Font, layoutProperties)) { // draw text drawingEngine.DrawText(data.Text, drawingFont, fontBrush, textRectangle, layoutProperties); } } } } } /// <summary> /// Returns the bounding box of specified polygon. /// </summary> /// <param name="points">The polygon points.</param> /// <returns>Bounding box of polygon.</returns> private static System.Drawing.RectangleF GetBoundingBox( System.Collections.Generic.IList<System.Drawing.PointF> points) { if (points.Count == 0) return System.Drawing.RectangleF.Empty; float x1, x2, y1, y2; x1 = x2 = points[0].X; y1 = y2 = points[0].Y; for (int i = 1; i < points.Count; i++) { float x = points[i].X; float y = points[i].Y; if (x1 > x) x1 = x; if (x2 < x) x2 = x; if (y1 > y) y1 = y; if (y2 < y) y2 = y; } return new System.Drawing.RectangleF(x1, y1, x2 - x1, y2 - y1); } #endregion } #endregion #region TextPolygonAnnotationView /// <summary> /// Determines how to display the annotation that displays polygon with text. /// </summary> public class TextPolygonAnnotationView : Vintasoft.Imaging.Annotation.UI.PolygonAnnotationView { #region Constructors /// <summary> /// Initializes a new instance of the <see cref="TextPolygonAnnotationView"/> class. /// </summary> /// <param name="annotationData">Object that stores the annotation data.</param> public TextPolygonAnnotationView(TextPolygonAnnotationData annotationData) : base(annotationData) { } #endregion #region Properties /// <summary> /// Gets or sets the text of the annotation. /// </summary> [System.ComponentModel.Description("Text of the annotation.")] public string Text { get { return TextAnnoData.Text; } set { TextAnnoData.Text = value; } } /// <summary> /// Gets or sets the <see cref="Font"/> of the annotation. /// </summary> [System.ComponentModel.Description("Text font of the annotation.")] public Vintasoft.Imaging.Annotation.AnnotationFont Font { get { return TextAnnoData.Font; } set { TextAnnoData.Font = value; } } /// <summary> /// Gets or sets the font brush of the annotation. /// </summary> [System.ComponentModel.Description("Font brush of the annotation.")] public Vintasoft.Imaging.Annotation.AnnotationBrushBase FontBrush { get { return TextAnnoData.FontBrush; } set { TextAnnoData.FontBrush = value; } } /// <summary> /// Gets typed annotation data value. /// </summary> TextPolygonAnnotationData TextAnnoData { get { return (TextPolygonAnnotationData)Data; } } #endregion #region Methods /// <summary> /// Creates a new object that is a copy of the current /// <see cref="Vintasoft.Imaging.Annotation.UI.TextAnnotationView"/> instance. /// </summary> /// <returns>A new object that is a copy of this /// <see cref="Vintasoft.Imaging.Annotation.UI.TextAnnotationView"/> instance.</returns> public override object Clone() { return new TextPolygonAnnotationView((TextPolygonAnnotationData)(Data.Clone())); } #endregion } #endregion
System.Object
 Vintasoft.Imaging.Annotation.UI.AnnotationView
   Vintasoft.Imaging.Annotation.UI.LineAnnotationViewBase
     Vintasoft.Imaging.Annotation.UI.LinesAnnotationView
       Vintasoft.Imaging.Annotation.UI.PolygonAnnotationView
Целевые платформы: .NET 8; .NET 7; .NET 6; .NET Framework 4.8, 4.7, 4.6, 4.5, 4.0, 3.5