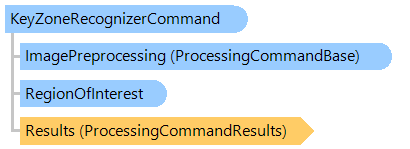
public abstract class KeyZoneRecognizerCommand : Vintasoft.Imaging.ImageProcessing.ProcessingCommandWithRegion
public __gc abstract class KeyZoneRecognizerCommand : public Vintasoft.Imaging.ImageProcessing.ProcessingCommandWithRegion*
public ref class KeyZoneRecognizerCommand abstract : public Vintasoft.Imaging.ImageProcessing.ProcessingCommandWithRegion^
'Declaration Public MustInherit Class KeyZoneRecognizerCommand Inherits Vintasoft.Imaging.ImageProcessing.ProcessingCommandWithRegion
Вот C#/VB.NET код, который демонстрирует, как создать собственный распознаватель зоны клавиш на основе распознавания штрих-кода.
''' <summary> ''' Represents key zone based on recognized barcode. ''' </summary> Public Class BarcodeKeyZone Inherits Vintasoft.Imaging.FormsProcessing.TemplateMatching.KeyZone #Region "Constructors" ''' <summary> ''' Initializes a new instance of the <see cref="BarcodeKeyZone"/> class. ''' </summary> ''' <param name="barcodeInfo">The barcode information.</param> Public Sub New(barcodeInfo As Vintasoft.Barcode.IBarcodeInfo) MyBase.New() _barcodeInfo = barcodeInfo If TypeOf barcodeInfo Is Vintasoft.Barcode.BarcodeInfo.AztecInfo Then ' get the barcode center from barcode info Dim bulleyeCenter As Vintasoft.Primitives.VintasoftPointF = DirectCast(barcodeInfo, Vintasoft.Barcode.BarcodeInfo.AztecInfo).BulleyeCenter _location = New System.Drawing.PointF(bulleyeCenter.X, bulleyeCenter.Y) Else ' calculate the barcode center _location = System.Drawing.PointF.Empty Dim points As Vintasoft.Primitives.VintasoftPointI() = barcodeInfo.Region.GetPoints() For i As Integer = 0 To points.Length - 1 _location.X += points(i).X _location.Y += points(i).Y Next _location.X /= points.Length _location.Y /= points.Length End If End Sub ''' <summary> ''' Initializes a new instance of the <see cref="BarcodeKeyZone"/> class. ''' </summary> ''' <param name="barcodeInfo">The barcode information.</param> ''' <param name="location">The location.</param> Private Sub New(barcodeInfo As Vintasoft.Barcode.IBarcodeInfo, location As System.Drawing.PointF) MyBase.New() _barcodeInfo = barcodeInfo _location = location End Sub #End Region #Region "Properties" Private _location As System.Drawing.PointF ''' <summary> ''' Gets the location of the key zone on the image. ''' </summary> Public Overrides ReadOnly Property Location() As System.Drawing.PointF Get Return _location End Get End Property Private _barcodeInfo As Vintasoft.Barcode.IBarcodeInfo ''' <summary> ''' Gets the barcode information. ''' </summary> Public ReadOnly Property BarcodeInfo() As Vintasoft.Barcode.IBarcodeInfo Get Return _barcodeInfo End Get End Property #End Region #Region "Methods" ''' <summary> ''' Applies a transformation to the key zone. ''' </summary> ''' <param name="m">The <see cref="Vintasoft.Imaging.AffineMatrix" /> ''' that specifies the transformation to apply.</param> Public Overrides Sub Transform(m As Vintasoft.Imaging.AffineMatrix) If m Is Nothing Then Return End If _location = Vintasoft.Imaging.PointFAffineTransform.TransformPoint(m, _location) End Sub ''' <summary> ''' Returns the similarity of specified <see cref="Vintasoft.Imaging.FormsProcessing.TemplateMatching.KeyZone" /> ''' and the current key zone. ''' </summary> ''' <param name="zone">The <see cref="Vintasoft.Imaging.FormsProcessing.TemplateMatching.KeyZone" /> ''' to compare with.</param> ''' <returns> ''' The similarity of specified keyzone and the current key zone in range from 0 to 1.<br /> ''' 0 means that zones are absolutely NOT similar; ''' 1 means that zones are perfectly similar. ''' </returns> Public Overrides Function CalculateSimilarity(zone As Vintasoft.Imaging.FormsProcessing.TemplateMatching.KeyZone) As Double Dim barcodeZone As BarcodeKeyZone = TryCast(zone, BarcodeKeyZone) If barcodeZone Is Nothing Then Return 0 End If If barcodeZone.BarcodeInfo.Value = BarcodeInfo.Value AndAlso barcodeZone.BarcodeInfo.BarcodeType = BarcodeInfo.BarcodeType Then Return 1 End If Return 0 End Function ''' <summary> ''' Creates a new object that is a copy of the current instance. ''' </summary> ''' <returns>A new object that is a copy of this instance.</returns> Public Overrides Function Clone() As Object Return New BarcodeKeyZone(BarcodeInfo, Location) End Function #End Region End Class ''' <summary> ''' Recognizes key zones, based on barcodes, on an image. ''' </summary> Public Class BarcodeKeyZoneRecognizerCommand Inherits Vintasoft.Imaging.FormsProcessing.TemplateMatching.KeyZoneRecognizerCommand #Region "Constructors" ''' <summary> ''' Initializes a new instance of the <see cref="BarcodeKeyZoneRecognizerCommand"/> class. ''' </summary> Public Sub New() MyBase.New() ' set default barcode reader settings Settings.ScanBarcodeTypes = Vintasoft.Barcode.BarcodeType.Aztec Settings.ScanDirection = Vintasoft.Barcode.ScanDirection.Horizontal Or Vintasoft.Barcode.ScanDirection.Vertical Settings.AutomaticRecognition = True ' 3 barcode by page Settings.ExpectedBarcodes = 3 End Sub #End Region #Region "Properties" Shared _supportedNativePixelFormats As System.Collections.ObjectModel.ReadOnlyCollection(Of Vintasoft.Imaging.PixelFormat) ''' <summary> ''' Gets a list of supported native pixel formats for this processing command. ''' </summary> Public Overrides ReadOnly Property SupportedNativePixelFormats() As System.Collections.ObjectModel.ReadOnlyCollection(Of Vintasoft.Imaging.PixelFormat) Get If _supportedNativePixelFormats Is Nothing Then Dim supportedNativePixelFormats__1 As New System.Collections.Generic.List(Of Vintasoft.Imaging.PixelFormat)() supportedNativePixelFormats__1.Add(Vintasoft.Imaging.PixelFormat.BlackWhite) supportedNativePixelFormats__1.Add(Vintasoft.Imaging.PixelFormat.Bgr24) supportedNativePixelFormats__1.Add(Vintasoft.Imaging.PixelFormat.Bgr32) supportedNativePixelFormats__1.Add(Vintasoft.Imaging.PixelFormat.Indexed1) supportedNativePixelFormats__1.Add(Vintasoft.Imaging.PixelFormat.Indexed8) supportedNativePixelFormats__1.Add(Vintasoft.Imaging.PixelFormat.Gray8) _supportedNativePixelFormats = supportedNativePixelFormats__1.AsReadOnly() End If Return _supportedNativePixelFormats End Get End Property Private _settings As New Vintasoft.Barcode.ReaderSettings() ''' <summary> ''' Gets or sets the barcode reader settings. ''' </summary> ''' <value> ''' The reader settings. ''' </value> Public Property Settings() As Vintasoft.Barcode.ReaderSettings Get Return _settings End Get Set If value Is Nothing Then Throw New System.ArgumentNullException() End If _settings = value End Set End Property #End Region #Region "Methods" ''' <summary> ''' Creates a new <see cref="BarcodeKeyZoneRecognizerCommand"/> that is a copy of the current ''' instance. ''' </summary> ''' <returns>A new <see cref="BarcodeKeyZoneRecognizerCommand"/> that is a copy of this ''' instance.</returns> Public Overrides Function Clone() As Object Dim recognizer As New BarcodeKeyZoneRecognizerCommand() recognizer.IsNested = IsNested recognizer.RegionOfInterest = RegionOfInterest recognizer.Settings = Settings.Clone() Return recognizer End Function ''' <summary> ''' Recognizes key zones in the specified rectangle of the specified image. ''' </summary> ''' <param name="image">The image where key zones must be searched.</param> ''' <param name="rect">The region of interest on image.</param> ''' <returns>An array of recognized key zones.</returns> Protected Overrides Function Recognize(image As Vintasoft.Imaging.VintasoftImage, rect As System.Drawing.Rectangle) As Vintasoft.Imaging.FormsProcessing.TemplateMatching.KeyZone() Dim reader As New Vintasoft.Barcode.BarcodeReader() reader.Settings = Settings.Clone() reader.Settings.ScanRectangle = New Vintasoft.Primitives.VintasoftRectI(rect.X, rect.Y, rect.Width, rect.Height) Using bitmap As Vintasoft.Imaging.VintasoftBitmap = image.GetAsVintasoftBitmap() Dim infos As Vintasoft.Barcode.IBarcodeInfo() = reader.ReadBarcodes(bitmap) Dim result As Vintasoft.Imaging.FormsProcessing.TemplateMatching.KeyZone() = New Vintasoft.Imaging.FormsProcessing.TemplateMatching.KeyZone(infos.Length - 1) {} For i As Integer = 0 To infos.Length - 1 result(i) = New BarcodeKeyZone(infos(i)) Next Return result End Using End Function #End Region End Class
/// <summary> /// Represents key zone based on recognized barcode. /// </summary> public class BarcodeKeyZone : Vintasoft.Imaging.FormsProcessing.TemplateMatching.KeyZone { #region Constructors /// <summary> /// Initializes a new instance of the <see cref="BarcodeKeyZone"/> class. /// </summary> /// <param name="barcodeInfo">The barcode information.</param> public BarcodeKeyZone(Vintasoft.Barcode.IBarcodeInfo barcodeInfo) : base() { _barcodeInfo = barcodeInfo; if (barcodeInfo is Vintasoft.Barcode.BarcodeInfo.AztecInfo) { // get the barcode center from barcode info Vintasoft.Primitives.VintasoftPointF bulleyeCenter = ((Vintasoft.Barcode.BarcodeInfo.AztecInfo)barcodeInfo).BulleyeCenter; _location = new System.Drawing.PointF(bulleyeCenter.X, bulleyeCenter.Y); } else { // calculate the barcode center _location = System.Drawing.PointF.Empty; Vintasoft.Primitives.VintasoftPointI[] points = barcodeInfo.Region.GetPoints(); for (int i = 0; i < points.Length; i++) { _location.X += points[i].X; _location.Y += points[i].Y; } _location.X /= points.Length; _location.Y /= points.Length; } } /// <summary> /// Initializes a new instance of the <see cref="BarcodeKeyZone"/> class. /// </summary> /// <param name="barcodeInfo">The barcode information.</param> /// <param name="location">The location.</param> private BarcodeKeyZone(Vintasoft.Barcode.IBarcodeInfo barcodeInfo, System.Drawing.PointF location) : base() { _barcodeInfo = barcodeInfo; _location = location; } #endregion #region Properties System.Drawing.PointF _location; /// <summary> /// Gets the location of the key zone on the image. /// </summary> public override System.Drawing.PointF Location { get { return _location; } } Vintasoft.Barcode.IBarcodeInfo _barcodeInfo; /// <summary> /// Gets the barcode information. /// </summary> public Vintasoft.Barcode.IBarcodeInfo BarcodeInfo { get { return _barcodeInfo; } } #endregion #region Methods /// <summary> /// Applies a transformation to the key zone. /// </summary> /// <param name="m">The <see cref="Vintasoft.Imaging.AffineMatrix" /> /// that specifies the transformation to apply.</param> public override void Transform(Vintasoft.Imaging.AffineMatrix m) { if (m == null) return; _location = Vintasoft.Imaging.PointFAffineTransform.TransformPoint(m, _location); } /// <summary> /// Returns the similarity of specified <see cref="Vintasoft.Imaging.FormsProcessing.TemplateMatching.KeyZone" /> /// and the current key zone. /// </summary> /// <param name="zone">The <see cref="Vintasoft.Imaging.FormsProcessing.TemplateMatching.KeyZone" /> /// to compare with.</param> /// <returns> /// The similarity of specified keyzone and the current key zone in range from 0 to 1.<br /> /// 0 means that zones are absolutely NOT similar; /// 1 means that zones are perfectly similar. /// </returns> public override double CalculateSimilarity( Vintasoft.Imaging.FormsProcessing.TemplateMatching.KeyZone zone) { BarcodeKeyZone barcodeZone = zone as BarcodeKeyZone; if (barcodeZone == null) return 0; if (barcodeZone.BarcodeInfo.Value == BarcodeInfo.Value && barcodeZone.BarcodeInfo.BarcodeType == BarcodeInfo.BarcodeType) return 1; return 0; } /// <summary> /// Creates a new object that is a copy of the current instance. /// </summary> /// <returns>A new object that is a copy of this instance.</returns> public override object Clone() { return new BarcodeKeyZone(BarcodeInfo, Location); } #endregion } /// <summary> /// Recognizes key zones, based on barcodes, on an image. /// </summary> public class BarcodeKeyZoneRecognizerCommand : Vintasoft.Imaging.FormsProcessing.TemplateMatching.KeyZoneRecognizerCommand { #region Constructors /// <summary> /// Initializes a new instance of the <see cref="BarcodeKeyZoneRecognizerCommand"/> class. /// </summary> public BarcodeKeyZoneRecognizerCommand() : base() { // set default barcode reader settings Settings.ScanBarcodeTypes = Vintasoft.Barcode.BarcodeType.Aztec; Settings.ScanDirection = Vintasoft.Barcode.ScanDirection.Horizontal | Vintasoft.Barcode.ScanDirection.Vertical; Settings.AutomaticRecognition = true; // 3 barcode by page Settings.ExpectedBarcodes = 3; } #endregion #region Properties static System.Collections.ObjectModel.ReadOnlyCollection<Vintasoft.Imaging.PixelFormat> _supportedNativePixelFormats; /// <summary> /// Gets a list of supported native pixel formats for this processing command. /// </summary> public override System.Collections.ObjectModel.ReadOnlyCollection<Vintasoft.Imaging.PixelFormat> SupportedNativePixelFormats { get { if (_supportedNativePixelFormats == null) { System.Collections.Generic.List<Vintasoft.Imaging.PixelFormat> supportedNativePixelFormats = new System.Collections.Generic.List<Vintasoft.Imaging.PixelFormat>(); supportedNativePixelFormats.Add(Vintasoft.Imaging.PixelFormat.BlackWhite); supportedNativePixelFormats.Add(Vintasoft.Imaging.PixelFormat.Bgr24); supportedNativePixelFormats.Add(Vintasoft.Imaging.PixelFormat.Bgr32); supportedNativePixelFormats.Add(Vintasoft.Imaging.PixelFormat.Indexed1); supportedNativePixelFormats.Add(Vintasoft.Imaging.PixelFormat.Indexed8); supportedNativePixelFormats.Add(Vintasoft.Imaging.PixelFormat.Gray8); _supportedNativePixelFormats = supportedNativePixelFormats.AsReadOnly(); } return _supportedNativePixelFormats; } } Vintasoft.Barcode.ReaderSettings _settings = new Vintasoft.Barcode.ReaderSettings(); /// <summary> /// Gets or sets the barcode reader settings. /// </summary> /// <value> /// The reader settings. /// </value> public Vintasoft.Barcode.ReaderSettings Settings { get { return _settings; } set { if (value == null) throw new System.ArgumentNullException(); _settings = value; } } #endregion #region Methods /// <summary> /// Creates a new <see cref="BarcodeKeyZoneRecognizerCommand"/> that is a copy of the current /// instance. /// </summary> /// <returns>A new <see cref="BarcodeKeyZoneRecognizerCommand"/> that is a copy of this /// instance.</returns> public override object Clone() { BarcodeKeyZoneRecognizerCommand recognizer = new BarcodeKeyZoneRecognizerCommand(); recognizer.IsNested = IsNested; recognizer.RegionOfInterest = RegionOfInterest; recognizer.Settings = Settings.Clone(); return recognizer; } /// <summary> /// Recognizes key zones in the specified rectangle of the specified image. /// </summary> /// <param name="image">The image where key zones must be searched.</param> /// <param name="rect">The region of interest on image.</param> /// <returns>An array of recognized key zones.</returns> protected override Vintasoft.Imaging.FormsProcessing.TemplateMatching.KeyZone[] Recognize( Vintasoft.Imaging.VintasoftImage image, System.Drawing.Rectangle rect) { Vintasoft.Barcode.BarcodeReader reader = new Vintasoft.Barcode.BarcodeReader(); reader.Settings = Settings.Clone(); reader.Settings.ScanRectangle = new Vintasoft.Primitives.VintasoftRectI(rect.X, rect.Y, rect.Width, rect.Height); using (Vintasoft.Imaging.VintasoftBitmap bitmap = image.GetAsVintasoftBitmap()) { Vintasoft.Barcode.IBarcodeInfo[] infos = reader.ReadBarcodes(bitmap); Vintasoft.Imaging.FormsProcessing.TemplateMatching.KeyZone[] result = new Vintasoft.Imaging.FormsProcessing.TemplateMatching.KeyZone[infos.Length]; for (int i = 0; i < infos.Length; i++) result[i] = new BarcodeKeyZone(infos[i]); return result; } } #endregion }
System.Object
Vintasoft.Imaging.ImageProcessing.ProcessingCommandBase
Vintasoft.Imaging.ImageProcessing.ProcessingCommandWithRegion
Vintasoft.Imaging.FormsProcessing.TemplateMatching.KeyZoneRecognizerCommand
Vintasoft.Imaging.FormsProcessing.TemplateMatching.KeyLineRecognizerCommand
Vintasoft.Imaging.FormsProcessing.TemplateMatching.KeyMarkRecognizerCommand
Целевые платформы: .NET 9; .NET 8; .NET 7; .NET 6; .NET Framework 4.8, 4.7, 4.6, 4.5, 4.0, 3.5
Члены типа KeyZoneRecognizerCommand
Пространство имен Vintasoft.Imaging.FormsProcessing.TemplateMatching