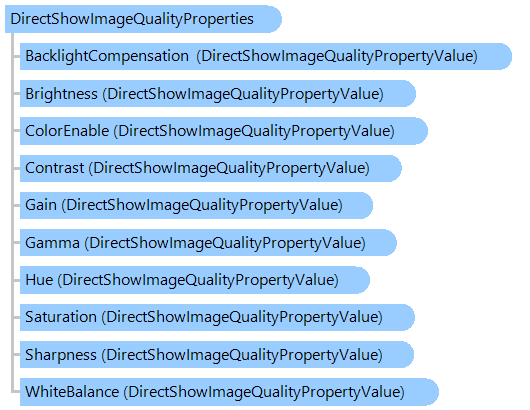
'Declaration Public Class DirectShowImageQualityProperties
public class DirectShowImageQualityProperties
public __gc class DirectShowImageQualityProperties
public ref class DirectShowImageQualityProperties
Вот C#/VB.NET код, который демонстрирует, как получить и установить свойства качества изображения камеры DirectShow:
''' <summary> ''' Gets and sets the image quality properties of DirectShow camera. ''' </summary> Public Shared Sub GetAndSetImageQualityProperties() ' get a list of installed cameras Dim captureDevices As System.Collections.ObjectModel.ReadOnlyCollection(Of Vintasoft.Imaging.Media.ImageCaptureDevice) = Vintasoft.Imaging.Media.ImageCaptureDeviceConfiguration.GetCaptureDevices() ' if cameras are not found If captureDevices.Count = 0 Then System.Console.WriteLine("No connected devices.") Return End If ' get the first available camera Dim camera As Vintasoft.Imaging.Media.DirectShowCamera = DirectCast(captureDevices(0), Vintasoft.Imaging.Media.DirectShowCamera) ' output camera name System.Console.WriteLine(camera.FriendlyName) Dim propertyValue As Vintasoft.Imaging.Media.DirectShowImageQualityPropertyValue Dim minValue As Integer, maxValue As Integer, [step] As Integer, defaultValue As Integer System.Console.WriteLine(" - BacklightCompensation") Try ' get supported values camera.ImageQuality.GetSupportedBacklightCompensationValues(minValue, maxValue, [step], defaultValue) System.Console.WriteLine(String.Format(" - Supported values: Min={0}, Max={1}, Step={2}, Default={3}", minValue, maxValue, [step], defaultValue)) ' get current value propertyValue = camera.ImageQuality.BacklightCompensation System.Console.WriteLine(String.Format(" - Current value: {0}", propertyValue.Value)) ' set current value camera.ImageQuality.BacklightCompensation = propertyValue Catch ex As System.Exception System.Console.WriteLine(" {0}", ex.Message) End Try System.Console.WriteLine(" - Brightness") Try ' get supported values camera.ImageQuality.GetSupportedBrightnessValues(minValue, maxValue, [step], defaultValue) System.Console.WriteLine(String.Format(" - Supported values: Min={0}, Max={1}, Step={2}, Default={3}", minValue, maxValue, [step], defaultValue)) ' get current value propertyValue = camera.ImageQuality.Brightness System.Console.WriteLine(String.Format(" - Current value: {0}", propertyValue.Value)) ' set current value camera.ImageQuality.Brightness = propertyValue Catch ex As System.Exception System.Console.WriteLine(" {0}", ex.Message) End Try System.Console.WriteLine(" - ColorEnable") Try ' get supported values camera.ImageQuality.GetSupportedColorEnableValues(minValue, maxValue, [step], defaultValue) System.Console.WriteLine(String.Format(" - Supported values: Min={0}, Max={1}, Step={2}, Default={3}", minValue, maxValue, [step], defaultValue)) ' get current value propertyValue = camera.ImageQuality.ColorEnable System.Console.WriteLine(String.Format(" - Current value: {0}", propertyValue.Value)) ' set current value camera.ImageQuality.ColorEnable = propertyValue Catch ex As System.Exception System.Console.WriteLine(" {0}", ex.Message) End Try System.Console.WriteLine(" - Contrast") Try ' get supported values camera.ImageQuality.GetSupportedContrastValues(minValue, maxValue, [step], defaultValue) System.Console.WriteLine(String.Format(" - Supported values: Min={0}, Max={1}, Step={2}, Default={3}", minValue, maxValue, [step], defaultValue)) ' get current value propertyValue = camera.ImageQuality.Contrast System.Console.WriteLine(String.Format(" - Current value: {0}", propertyValue.Value)) ' set current value camera.ImageQuality.Contrast = propertyValue Catch ex As System.Exception System.Console.WriteLine(" {0}", ex.Message) End Try System.Console.WriteLine(" - Gain") Try ' get supported values camera.ImageQuality.GetSupportedGainValues(minValue, maxValue, [step], defaultValue) System.Console.WriteLine(String.Format(" - Supported values: Min={0}, Max={1}, Step={2}, Default={3}", minValue, maxValue, [step], defaultValue)) ' get current value propertyValue = camera.ImageQuality.Gain System.Console.WriteLine(String.Format(" - Current value: {0}", propertyValue.Value)) ' set current value camera.ImageQuality.Gain = propertyValue Catch ex As System.Exception System.Console.WriteLine(" {0}", ex.Message) End Try System.Console.WriteLine(" - Gamma") Try ' get supported values camera.ImageQuality.GetSupportedGammaValues(minValue, maxValue, [step], defaultValue) System.Console.WriteLine(String.Format(" - Supported values: Min={0}, Max={1}, Step={2}, Default={3}", minValue, maxValue, [step], defaultValue)) ' get current value propertyValue = camera.ImageQuality.Gamma System.Console.WriteLine(String.Format(" - Current value: {0}", propertyValue.Value)) ' set current value camera.ImageQuality.Gamma = propertyValue Catch ex As System.Exception System.Console.WriteLine(" {0}", ex.Message) End Try System.Console.WriteLine(" - Hue") Try ' get supported values camera.ImageQuality.GetSupportedHueValues(minValue, maxValue, [step], defaultValue) System.Console.WriteLine(String.Format(" - Supported values: Min={0}, Max={1}, Step={2}, Default={3}", minValue, maxValue, [step], defaultValue)) ' get current value propertyValue = camera.ImageQuality.Hue System.Console.WriteLine(String.Format(" - Current value: {0}", propertyValue.Value)) ' set current value camera.ImageQuality.Hue = propertyValue Catch ex As System.Exception System.Console.WriteLine(" {0}", ex.Message) End Try System.Console.WriteLine(" - Saturation") Try ' get supported values camera.ImageQuality.GetSupportedSaturationValues(minValue, maxValue, [step], defaultValue) System.Console.WriteLine(String.Format(" - Supported values: Min={0}, Max={1}, Step={2}, Default={3}", minValue, maxValue, [step], defaultValue)) ' get current value propertyValue = camera.ImageQuality.Saturation System.Console.WriteLine(String.Format(" - Current value: {0}", propertyValue.Value)) ' set current value camera.ImageQuality.Saturation = propertyValue Catch ex As System.Exception System.Console.WriteLine(" {0}", ex.Message) End Try System.Console.WriteLine(" - Sharpness") Try ' get supported values camera.ImageQuality.GetSupportedSharpnessValues(minValue, maxValue, [step], defaultValue) System.Console.WriteLine(String.Format(" - Supported values: Min={0}, Max={1}, Step={2}, Default={3}", minValue, maxValue, [step], defaultValue)) ' get current value propertyValue = camera.ImageQuality.Sharpness System.Console.WriteLine(String.Format(" - Current value: {0}", propertyValue.Value)) ' set current value camera.ImageQuality.Sharpness = propertyValue Catch ex As System.Exception System.Console.WriteLine(" {0}", ex.Message) End Try System.Console.WriteLine(" - WhiteBalance") Try ' get supported values camera.ImageQuality.GetSupportedWhiteBalanceValues(minValue, maxValue, [step], defaultValue) System.Console.WriteLine(String.Format(" - Supported values: Min={0}, Max={1}, Step={2}, Default={3}", minValue, maxValue, [step], defaultValue)) ' get current value propertyValue = camera.ImageQuality.WhiteBalance System.Console.WriteLine(String.Format(" - Current value: {0}", propertyValue.Value)) ' set current value camera.ImageQuality.WhiteBalance = propertyValue Catch ex As System.Exception System.Console.WriteLine(" {0}", ex.Message) End Try End Sub
/// <summary> /// Gets and sets the image quality properties of DirectShow camera. /// </summary> public static void GetAndSetImageQualityProperties() { // get a list of installed cameras System.Collections.ObjectModel.ReadOnlyCollection<Vintasoft.Imaging.Media.ImageCaptureDevice> captureDevices = Vintasoft.Imaging.Media.ImageCaptureDeviceConfiguration.GetCaptureDevices(); // if cameras are not found if (captureDevices.Count == 0) { System.Console.WriteLine("No connected devices."); return; } // get the first available camera Vintasoft.Imaging.Media.DirectShowCamera camera = (Vintasoft.Imaging.Media.DirectShowCamera)captureDevices[0]; // output camera name System.Console.WriteLine(camera.FriendlyName); Vintasoft.Imaging.Media.DirectShowImageQualityPropertyValue propertyValue; int minValue, maxValue, step, defaultValue; System.Console.WriteLine(" - BacklightCompensation"); try { // get supported values camera.ImageQuality.GetSupportedBacklightCompensationValues(out minValue, out maxValue, out step, out defaultValue); System.Console.WriteLine(string.Format(" - Supported values: Min={0}, Max={1}, Step={2}, Default={3}", minValue, maxValue, step, defaultValue)); // get current value propertyValue = camera.ImageQuality.BacklightCompensation; System.Console.WriteLine(string.Format(" - Current value: {0}", propertyValue.Value)); // set current value camera.ImageQuality.BacklightCompensation = propertyValue; } catch (System.Exception ex) { System.Console.WriteLine(" {0}", ex.Message); } System.Console.WriteLine(" - Brightness"); try { // get supported values camera.ImageQuality.GetSupportedBrightnessValues(out minValue, out maxValue, out step, out defaultValue); System.Console.WriteLine(string.Format(" - Supported values: Min={0}, Max={1}, Step={2}, Default={3}", minValue, maxValue, step, defaultValue)); // get current value propertyValue = camera.ImageQuality.Brightness; System.Console.WriteLine(string.Format(" - Current value: {0}", propertyValue.Value)); // set current value camera.ImageQuality.Brightness = propertyValue; } catch (System.Exception ex) { System.Console.WriteLine(" {0}", ex.Message); } System.Console.WriteLine(" - ColorEnable"); try { // get supported values camera.ImageQuality.GetSupportedColorEnableValues(out minValue, out maxValue, out step, out defaultValue); System.Console.WriteLine(string.Format(" - Supported values: Min={0}, Max={1}, Step={2}, Default={3}", minValue, maxValue, step, defaultValue)); // get current value propertyValue = camera.ImageQuality.ColorEnable; System.Console.WriteLine(string.Format(" - Current value: {0}", propertyValue.Value)); // set current value camera.ImageQuality.ColorEnable = propertyValue; } catch (System.Exception ex) { System.Console.WriteLine(" {0}", ex.Message); } System.Console.WriteLine(" - Contrast"); try { // get supported values camera.ImageQuality.GetSupportedContrastValues(out minValue, out maxValue, out step, out defaultValue); System.Console.WriteLine(string.Format(" - Supported values: Min={0}, Max={1}, Step={2}, Default={3}", minValue, maxValue, step, defaultValue)); // get current value propertyValue = camera.ImageQuality.Contrast; System.Console.WriteLine(string.Format(" - Current value: {0}", propertyValue.Value)); // set current value camera.ImageQuality.Contrast = propertyValue; } catch (System.Exception ex) { System.Console.WriteLine(" {0}", ex.Message); } System.Console.WriteLine(" - Gain"); try { // get supported values camera.ImageQuality.GetSupportedGainValues(out minValue, out maxValue, out step, out defaultValue); System.Console.WriteLine(string.Format(" - Supported values: Min={0}, Max={1}, Step={2}, Default={3}", minValue, maxValue, step, defaultValue)); // get current value propertyValue = camera.ImageQuality.Gain; System.Console.WriteLine(string.Format(" - Current value: {0}", propertyValue.Value)); // set current value camera.ImageQuality.Gain = propertyValue; } catch (System.Exception ex) { System.Console.WriteLine(" {0}", ex.Message); } System.Console.WriteLine(" - Gamma"); try { // get supported values camera.ImageQuality.GetSupportedGammaValues(out minValue, out maxValue, out step, out defaultValue); System.Console.WriteLine(string.Format(" - Supported values: Min={0}, Max={1}, Step={2}, Default={3}", minValue, maxValue, step, defaultValue)); // get current value propertyValue = camera.ImageQuality.Gamma; System.Console.WriteLine(string.Format(" - Current value: {0}", propertyValue.Value)); // set current value camera.ImageQuality.Gamma = propertyValue; } catch (System.Exception ex) { System.Console.WriteLine(" {0}", ex.Message); } System.Console.WriteLine(" - Hue"); try { // get supported values camera.ImageQuality.GetSupportedHueValues(out minValue, out maxValue, out step, out defaultValue); System.Console.WriteLine(string.Format(" - Supported values: Min={0}, Max={1}, Step={2}, Default={3}", minValue, maxValue, step, defaultValue)); // get current value propertyValue = camera.ImageQuality.Hue; System.Console.WriteLine(string.Format(" - Current value: {0}", propertyValue.Value)); // set current value camera.ImageQuality.Hue = propertyValue; } catch (System.Exception ex) { System.Console.WriteLine(" {0}", ex.Message); } System.Console.WriteLine(" - Saturation"); try { // get supported values camera.ImageQuality.GetSupportedSaturationValues(out minValue, out maxValue, out step, out defaultValue); System.Console.WriteLine(string.Format(" - Supported values: Min={0}, Max={1}, Step={2}, Default={3}", minValue, maxValue, step, defaultValue)); // get current value propertyValue = camera.ImageQuality.Saturation; System.Console.WriteLine(string.Format(" - Current value: {0}", propertyValue.Value)); // set current value camera.ImageQuality.Saturation = propertyValue; } catch (System.Exception ex) { System.Console.WriteLine(" {0}", ex.Message); } System.Console.WriteLine(" - Sharpness"); try { // get supported values camera.ImageQuality.GetSupportedSharpnessValues(out minValue, out maxValue, out step, out defaultValue); System.Console.WriteLine(string.Format(" - Supported values: Min={0}, Max={1}, Step={2}, Default={3}", minValue, maxValue, step, defaultValue)); // get current value propertyValue = camera.ImageQuality.Sharpness; System.Console.WriteLine(string.Format(" - Current value: {0}", propertyValue.Value)); // set current value camera.ImageQuality.Sharpness = propertyValue; } catch (System.Exception ex) { System.Console.WriteLine(" {0}", ex.Message); } System.Console.WriteLine(" - WhiteBalance"); try { // get supported values camera.ImageQuality.GetSupportedWhiteBalanceValues(out minValue, out maxValue, out step, out defaultValue); System.Console.WriteLine(string.Format(" - Supported values: Min={0}, Max={1}, Step={2}, Default={3}", minValue, maxValue, step, defaultValue)); // get current value propertyValue = camera.ImageQuality.WhiteBalance; System.Console.WriteLine(string.Format(" - Current value: {0}", propertyValue.Value)); // set current value camera.ImageQuality.WhiteBalance = propertyValue; } catch (System.Exception ex) { System.Console.WriteLine(" {0}", ex.Message); } }
System.Object
 Vintasoft.Imaging.Media.DirectShowImageQualityProperties
Целевые платформы: .NET 8; .NET 7; .NET 6; .NET Framework 4.8, 4.7, 4.6, 4.5, 4.0, 3.5