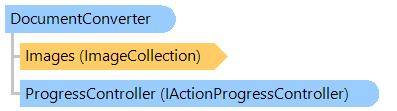
public class DocumentConverter
public __gc class DocumentConverter
public ref class DocumentConverter
'Declaration Public Class DocumentConverter
Используйте событие ImageDecodingStarting для установки параметров рендеринга или декодирования входного изображения.
Используйте событие ImageEncodingStarting для обработки вывода изображение перед кодированием или установите настройки кодировки выходного изображения.
Вот C#/VB.NET код, который демонстрирует, как преобразовать изображение документа:
''' <summary> ''' Converts a file from one format to another format. ''' </summary> ''' <param name="inFilename">The input filename.</param> ''' <param name="outFilename">The output filename.</param> Public Shared Sub Convert(inFilename As String, outFilename As String) ' create the document converter Using converter As New Vintasoft.Imaging.DocumentConverter() ' handle the ImageDecodingStarting event to set decoding setting of an input image AddHandler converter.ImageDecodingStarting, AddressOf Converter_ImageDecodingStarting ' handle the ImageEncodingStarting event to set encoding setting of an output image AddHandler converter.ImageEncodingStarting, AddressOf Converter_ImageEncodingStarting ' handle the conversion progress event AddHandler converter.ConversionProgress, AddressOf Converter_ConversionProgress ' allow multithreading converter.MaxThreads = System.Environment.ProcessorCount ' add input images to the document converter converter.Images.Add(inFilename) ' execute conversion converter.Convert(outFilename) ' clear resources converter.Images.ClearAndDisposeItems() End Using System.Console.WriteLine("Conversion is finished.") End Sub ''' <summary> ''' Handles the ConversionProgress event of the DocumentConverter. ''' </summary> ''' <param name="sender">The source of the event.</param> ''' <param name="e">The <see cref="Vintasoft.Imaging.ProgressEventArgs"/> instance containing the event data.</param> Private Shared Sub Converter_ConversionProgress(sender As Object, e As Vintasoft.Imaging.ProgressEventArgs) System.Console.WriteLine(String.Format("Conversion {0}%...", e.Progress)) End Sub ''' <summary> ''' Handles the ImageDecodingStarting event of the DocumentConverter. ''' </summary> ''' <param name="sender">The source of the event.</param> ''' <param name="e">The <see cref="Vintasoft.Imaging.ImageDecodingStartingEventArgs"/> instance containing the event data.</param> Private Shared Sub Converter_ImageDecodingStarting(sender As Object, e As Vintasoft.Imaging.ImageDecodingStartingEventArgs) ' if input file is PDF document If e.Image.SourceInfo.DecoderName = "Pdf" Then ' set the rendring resolution to 150 DPI for input PDF documents e.RenderingSettings = New Vintasoft.Imaging.Codecs.Decoders.RenderingSettings(New Vintasoft.Imaging.Resolution(150)) End If End Sub ''' <summary> ''' Handles the ImageEncodingStarting event of the DocumentConverter. ''' </summary> ''' <param name="sender">The source of the event.</param> ''' <param name="e">The <see cref="Vintasoft.Imaging.ImageEncodingStartingEventArgs"/> instance containing the event data.</param> Private Shared Sub Converter_ImageEncodingStarting(sender As Object, e As Vintasoft.Imaging.ImageEncodingStartingEventArgs) ' if output file is TIFF file If TypeOf e.Encoder Is Vintasoft.Imaging.Codecs.Encoders.TiffEncoder Then Dim tiffEncoder As Vintasoft.Imaging.Codecs.Encoders.TiffEncoder = DirectCast(e.Encoder, Vintasoft.Imaging.Codecs.Encoders.TiffEncoder) ' if image is black-white image If e.Image.BitsPerPixel = 1 Then ' use CCITT Group Fax 4 compression tiffEncoder.Settings.Compression = Vintasoft.Imaging.Codecs.ImageFiles.Tiff.TiffCompression.CcittGroup4 ' if image is grayscale- or RGB-image ElseIf e.Image.BitsPerPixel = 8 OrElse e.Image.BitsPerPixel = 24 Then ' use JPEG compression tiffEncoder.Settings.Compression = Vintasoft.Imaging.Codecs.ImageFiles.Tiff.TiffCompression.Jpeg tiffEncoder.Settings.JpegEncoderSettings.Quality = 70 Else ' use ZIP compression tiffEncoder.Settings.Compression = Vintasoft.Imaging.Codecs.ImageFiles.Tiff.TiffCompression.Zip End If ' if output file is PDF encoder ElseIf TypeOf e.Encoder Is Vintasoft.Imaging.Codecs.Encoders.PdfEncoder Then Dim pdfEncoder As Vintasoft.Imaging.Codecs.Encoders.PdfEncoder = DirectCast(e.Encoder, Vintasoft.Imaging.Codecs.Encoders.PdfEncoder) ' if image is black-white image If e.Image.BitsPerPixel = 1 Then ' use CCITT Group Fax 4 compression pdfEncoder.Settings.Compression = Vintasoft.Imaging.Codecs.Encoders.PdfImageCompression.CcittFax ' if image is grayscale- or RGB-image ElseIf e.Image.BitsPerPixel = 8 OrElse e.Image.BitsPerPixel = 24 Then ' use JPEG compression pdfEncoder.Settings.Compression = Vintasoft.Imaging.Codecs.Encoders.PdfImageCompression.Jpeg pdfEncoder.Settings.JpegSettings.Quality = 70 Else ' use ZIP compression pdfEncoder.Settings.Compression = Vintasoft.Imaging.Codecs.Encoders.PdfImageCompression.Zip End If End If End Sub
/// <summary> /// Converts a file from one format to another format. /// </summary> /// <param name="inFilename">The input filename.</param> /// <param name="outFilename">The output filename.</param> public static void Convert(string inFilename, string outFilename) { // create the document converter using (Vintasoft.Imaging.DocumentConverter converter = new Vintasoft.Imaging.DocumentConverter()) { // handle the ImageDecodingStarting event to set decoding setting of an input image converter.ImageDecodingStarting += Converter_ImageDecodingStarting; // handle the ImageEncodingStarting event to set encoding setting of an output image converter.ImageEncodingStarting += Converter_ImageEncodingStarting; // handle the conversion progress event converter.ConversionProgress += Converter_ConversionProgress; // allow multithreading converter.MaxThreads = System.Environment.ProcessorCount; // add input images to the document converter converter.Images.Add(inFilename); // execute conversion converter.Convert(outFilename); // clear resources converter.Images.ClearAndDisposeItems(); } System.Console.WriteLine("Conversion is finished."); } /// <summary> /// Handles the ConversionProgress event of the DocumentConverter. /// </summary> /// <param name="sender">The source of the event.</param> /// <param name="e">The <see cref="Vintasoft.Imaging.ProgressEventArgs"/> instance containing the event data.</param> private static void Converter_ConversionProgress(object sender, Vintasoft.Imaging.ProgressEventArgs e) { System.Console.WriteLine(string.Format("Conversion {0}%...", e.Progress)); } /// <summary> /// Handles the ImageDecodingStarting event of the DocumentConverter. /// </summary> /// <param name="sender">The source of the event.</param> /// <param name="e">The <see cref="Vintasoft.Imaging.ImageDecodingStartingEventArgs"/> instance containing the event data.</param> private static void Converter_ImageDecodingStarting(object sender, Vintasoft.Imaging.ImageDecodingStartingEventArgs e) { // if input file is PDF document if (e.Image.SourceInfo.DecoderName == "Pdf") // set the rendring resolution to 150 DPI for input PDF documents e.RenderingSettings = new Vintasoft.Imaging.Codecs.Decoders.RenderingSettings(new Vintasoft.Imaging.Resolution(150)); } /// <summary> /// Handles the ImageEncodingStarting event of the DocumentConverter. /// </summary> /// <param name="sender">The source of the event.</param> /// <param name="e">The <see cref="Vintasoft.Imaging.ImageEncodingStartingEventArgs"/> instance containing the event data.</param> private static void Converter_ImageEncodingStarting(object sender, Vintasoft.Imaging.ImageEncodingStartingEventArgs e) { // if output file is TIFF file if (e.Encoder is Vintasoft.Imaging.Codecs.Encoders.TiffEncoder) { Vintasoft.Imaging.Codecs.Encoders.TiffEncoder tiffEncoder = (Vintasoft.Imaging.Codecs.Encoders.TiffEncoder)e.Encoder; // if image is black-white image if (e.Image.BitsPerPixel == 1) { // use CCITT Group Fax 4 compression tiffEncoder.Settings.Compression = Vintasoft.Imaging.Codecs.ImageFiles.Tiff.TiffCompression.CcittGroup4; } // if image is grayscale- or RGB-image else if (e.Image.BitsPerPixel == 8 || e.Image.BitsPerPixel == 24) { // use JPEG compression tiffEncoder.Settings.Compression = Vintasoft.Imaging.Codecs.ImageFiles.Tiff.TiffCompression.Jpeg; tiffEncoder.Settings.JpegEncoderSettings.Quality = 70; } else { // use ZIP compression tiffEncoder.Settings.Compression = Vintasoft.Imaging.Codecs.ImageFiles.Tiff.TiffCompression.Zip; } } // if output file is PDF encoder else if (e.Encoder is Vintasoft.Imaging.Codecs.Encoders.PdfEncoder) { Vintasoft.Imaging.Codecs.Encoders.PdfEncoder pdfEncoder = (Vintasoft.Imaging.Codecs.Encoders.PdfEncoder)e.Encoder; // if image is black-white image if (e.Image.BitsPerPixel == 1) { // use CCITT Group Fax 4 compression pdfEncoder.Settings.Compression = Vintasoft.Imaging.Codecs.Encoders.PdfImageCompression.CcittFax; } // if image is grayscale- or RGB-image else if (e.Image.BitsPerPixel == 8 || e.Image.BitsPerPixel == 24) { // use JPEG compression pdfEncoder.Settings.Compression = Vintasoft.Imaging.Codecs.Encoders.PdfImageCompression.Jpeg; pdfEncoder.Settings.JpegSettings.Quality = 70; } else { // use ZIP compression pdfEncoder.Settings.Compression = Vintasoft.Imaging.Codecs.Encoders.PdfImageCompression.Zip; } } }
System.Object
Vintasoft.Imaging.DocumentConverter
Целевые платформы: .NET 9; .NET 8; .NET 7; .NET 6; .NET Framework 4.8, 4.7, 4.6, 4.5, 4.0, 3.5
Члены типа DocumentConverter
Пространство имен Vintasoft.Imaging
Images
MaxThreads
GetSinglePageFilename(String,VintasoftImage)