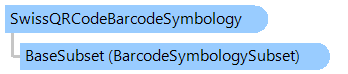
'Declaration Public Class SwissQRCodeBarcodeSymbology Inherits BarcodeSymbologySubset
public class SwissQRCodeBarcodeSymbology : BarcodeSymbologySubset
public __gc class SwissQRCodeBarcodeSymbology : public BarcodeSymbologySubset*
public ref class SwissQRCodeBarcodeSymbology : public BarcodeSymbologySubset^
Вот C#/VB.NET код, который демонстрирует, как сгенерировать и распознать штрих-код Swiss QR Code:
Class SwissQRCodeExample ''' <summary> ''' Generates and recognizes Swiss QR Code barcode. ''' </summary> Public Shared Sub Test() ' create Swiss QR Code Value Dim barcodeValue As New Vintasoft.Barcode.BarcodeInfo.SwissQRCodeValueItem() barcodeValue.IBAN = "CH4431999123000889012" barcodeValue.CreditorAddressType = "S" barcodeValue.CreditorName = "Robert Schneider AG" barcodeValue.CreditorStreetOrAddressLine1 = "Rue du Lac" barcodeValue.CreditorBuildingNumberOrAddressLine2 = "1268" barcodeValue.CreditorTown = "Biel" barcodeValue.CreditorCountry = "CH" barcodeValue.Amount = "1949.75" barcodeValue.AmountCurrency = "CHF" barcodeValue.UltimateDebtorAddressType = "S" barcodeValue.UltimateDebtorName = "Pia - Maria Rutschmann - Schnyder" barcodeValue.UltimateDebtorStreetOrAddressLine1 = "Grosse Marktgasse" barcodeValue.UltimateDebtorBuildingNumberOrAddressLine2 = "28" barcodeValue.UltimateDebtorTown = "Rorschach" barcodeValue.UltimateDebtorCountry = "CH" barcodeValue.PaymentReferenceType = "QRR" barcodeValue.PaymentReference = "210000000003139471430009017" barcodeValue.UnstructuredMessage = "Order of 15 June 2020" barcodeValue.BillInformation = "//S1/10/10201409/11/200701/20/140.000-53/30/102673831/31/200615/32/7.7/33/7.7:139.40/40/0:30" barcodeValue.AlternativeSchemeParameters1 = "Name AV1: UV; UltraPay005; 12345" barcodeValue.AlternativeSchemeParameters2 = "Name AV2: XY; XYService; 54321" ' generate barcode image Using barcodeImage As Vintasoft.Imaging.VintasoftBitmap = Generate(barcodeValue) ' recognize barcode from image Dim recognizedBarcode As Vintasoft.Barcode.BarcodeInfo.SwissQRCodeBarcodeInfo = Recognize(barcodeImage) ' if initial barcode value does NOT equal to the recognized barcode value If barcodeValue.ToString() <> recognizedBarcode.DecodedValue.ToString() Then Throw New System.ApplicationException("Initial barcode value does NOT equal to the recognized barcode value.") End If ' print recognized barcode value Dim sb As New System.Text.StringBuilder() Dim value As Vintasoft.Barcode.BarcodeInfo.SwissQRCodeValueItem = recognizedBarcode.DecodedValue sb.AppendLine(String.Format("Version: {0}", value.Version)) sb.AppendLine(String.Format("CodingType: {0}", value.CodingType)) If Not String.IsNullOrEmpty(value.IBAN) Then sb.AppendLine(String.Format("IBAN: {0}", value.IBAN)) End If If Not String.IsNullOrEmpty(value.CreditorAddressType) Then sb.AppendLine(String.Format("Creditor address type: {0}", value.CreditorAddressType)) End If If Not String.IsNullOrEmpty(value.CreditorName) Then sb.AppendLine(String.Format("Creditor name: {0}", value.CreditorName)) End If If Not String.IsNullOrEmpty(value.CreditorStreetOrAddressLine1) Then sb.AppendLine(String.Format("Creditor street or address line 1: {0}", value.CreditorStreetOrAddressLine1)) End If If Not String.IsNullOrEmpty(value.CreditorBuildingNumberOrAddressLine2) Then sb.AppendLine(String.Format("Creditor building number or address line 2: {0}", value.CreditorBuildingNumberOrAddressLine2)) End If If Not String.IsNullOrEmpty(value.CreditorTown) Then sb.AppendLine(String.Format("Creditor town: {0}", value.CreditorTown)) End If If Not String.IsNullOrEmpty(value.CreditorCountry) Then sb.AppendLine(String.Format("Creditor country: {0}", value.CreditorCountry)) End If If Not String.IsNullOrEmpty(value.Amount) Then sb.AppendLine(String.Format("Amount: {0}", value.Amount)) End If If Not String.IsNullOrEmpty(value.AmountCurrency) Then sb.AppendLine(String.Format("Amount currency: {0}", value.AmountCurrency)) End If If Not String.IsNullOrEmpty(value.UltimateDebtorAddressType) Then sb.AppendLine(String.Format("Ultimate debtor address type: {0}", value.UltimateDebtorAddressType)) End If If Not String.IsNullOrEmpty(value.UltimateDebtorName) Then sb.AppendLine(String.Format("Ultimate debtor name: {0}", value.UltimateDebtorName)) End If If Not String.IsNullOrEmpty(value.UltimateDebtorStreetOrAddressLine1) Then sb.AppendLine(String.Format("Ultimate debtor street or address line 1: {0}", value.UltimateDebtorStreetOrAddressLine1)) End If If Not String.IsNullOrEmpty(value.UltimateDebtorBuildingNumberOrAddressLine2) Then sb.AppendLine(String.Format("Ultimate debtor building number or address line 2: {0}", value.UltimateDebtorBuildingNumberOrAddressLine2)) End If If Not String.IsNullOrEmpty(value.UltimateDebtorTown) Then sb.AppendLine(String.Format("Ultimate debtor town: {0}", value.UltimateDebtorTown)) End If If Not String.IsNullOrEmpty(value.UltimateDebtorCountry) Then sb.AppendLine(String.Format("Ultimate debtor country: {0}", value.UltimateDebtorCountry)) End If If Not String.IsNullOrEmpty(value.PaymentReferenceType) Then sb.AppendLine(String.Format("Payment reference type: {0}", value.PaymentReferenceType)) End If If Not String.IsNullOrEmpty(value.PaymentReference) Then sb.AppendLine(String.Format("Payment reference: {0}", value.PaymentReference)) End If If Not String.IsNullOrEmpty(value.UnstructuredMessage) Then sb.AppendLine(String.Format("Unstructured message: {0}", value.UnstructuredMessage)) End If If Not String.IsNullOrEmpty(value.BillInformation) Then sb.AppendLine(String.Format("Bill information: {0}", value.BillInformation)) End If If Not String.IsNullOrEmpty(value.AlternativeSchemeParameters1) Then sb.AppendLine(String.Format("Alternative scheme parameters 1: {0}", value.AlternativeSchemeParameters1)) End If If Not String.IsNullOrEmpty(value.AlternativeSchemeParameters1) Then sb.AppendLine(String.Format("Alternative scheme parameters 2: {0}", value.AlternativeSchemeParameters2)) End If System.Console.WriteLine(sb.ToString()) End Using End Sub ''' <summary> ''' Generates the Swiss QR Code barcode image. ''' </summary> ''' <param name="barcodeValue">The barcode value.</param> Private Shared Function Generate(barcodeValue As Vintasoft.Barcode.BarcodeInfo.SwissQRCodeValueItem) As Vintasoft.Imaging.VintasoftBitmap ' create barcode writer Using writer As New Vintasoft.Barcode.BarcodeWriter() ' encode Swiss QR Code barcode to the writer settings Vintasoft.Barcode.SymbologySubsets.BarcodeSymbologySubsets.SwissQRCode.Encode(barcodeValue, writer.Settings) ' generate barcode image Return writer.GetBarcodeAsVintasoftBitmap() End Using End Function ''' <summary> ''' Recognizes the Swiss QR Code barcode from image. ''' </summary> ''' <param name="barcodeImage">The barcode image.</param> Private Shared Function Recognize(barcodeImage As Vintasoft.Imaging.VintasoftBitmap) As Vintasoft.Barcode.BarcodeInfo.SwissQRCodeBarcodeInfo ' create barcode reader Using reader As New Vintasoft.Barcode.BarcodeReader() ' specify that Swiss QR Code barcode must be recognized reader.Settings.ScanBarcodeSubsets.Add(Vintasoft.Barcode.SymbologySubsets.BarcodeSymbologySubsets.SwissQRCode) ' recognize barcodes in image Dim info As Vintasoft.Barcode.IBarcodeInfo = reader.ReadBarcodes(barcodeImage)(0) ' return information about recognized Swiss QR Code barcode Return DirectCast(info, Vintasoft.Barcode.BarcodeInfo.SwissQRCodeBarcodeInfo) End Using End Function End Class
class SwissQRCodeExample { /// <summary> /// Generates and recognizes Swiss QR Code barcode. /// </summary> public static void Test() { // create Swiss QR Code Value Vintasoft.Barcode.BarcodeInfo.SwissQRCodeValueItem barcodeValue = new Vintasoft.Barcode.BarcodeInfo.SwissQRCodeValueItem(); barcodeValue.IBAN= "CH4431999123000889012"; barcodeValue.CreditorAddressType = "S"; barcodeValue.CreditorName = "Robert Schneider AG"; barcodeValue.CreditorStreetOrAddressLine1 = "Rue du Lac"; barcodeValue.CreditorBuildingNumberOrAddressLine2 = "1268"; barcodeValue.CreditorTown = "Biel"; barcodeValue.CreditorCountry = "CH"; barcodeValue.Amount = "1949.75"; barcodeValue.AmountCurrency = "CHF"; barcodeValue.UltimateDebtorAddressType = "S"; barcodeValue.UltimateDebtorName = "Pia - Maria Rutschmann - Schnyder"; barcodeValue.UltimateDebtorStreetOrAddressLine1 = "Grosse Marktgasse"; barcodeValue.UltimateDebtorBuildingNumberOrAddressLine2 = "28"; barcodeValue.UltimateDebtorTown = "Rorschach"; barcodeValue.UltimateDebtorCountry = "CH"; barcodeValue.PaymentReferenceType = "QRR"; barcodeValue.PaymentReference = "210000000003139471430009017"; barcodeValue.UnstructuredMessage = "Order of 15 June 2020"; barcodeValue.BillInformation = "//S1/10/10201409/11/200701/20/140.000-53/30/102673831/31/200615/32/7.7/33/7.7:139.40/40/0:30"; barcodeValue.AlternativeSchemeParameters1 = "Name AV1: UV; UltraPay005; 12345"; barcodeValue.AlternativeSchemeParameters2 = "Name AV2: XY; XYService; 54321"; // generate barcode image using (Vintasoft.Imaging.VintasoftBitmap barcodeImage = Generate(barcodeValue)) { // recognize barcode from image Vintasoft.Barcode.BarcodeInfo.SwissQRCodeBarcodeInfo recognizedBarcode = Recognize(barcodeImage); // if initial barcode value does NOT equal to the recognized barcode value if (barcodeValue.ToString() != recognizedBarcode.DecodedValue.ToString()) throw new System.ApplicationException("Initial barcode value does NOT equal to the recognized barcode value."); // print recognized barcode value System.Text.StringBuilder sb = new System.Text.StringBuilder(); Vintasoft.Barcode.BarcodeInfo.SwissQRCodeValueItem value = recognizedBarcode.DecodedValue; sb.AppendLine(string.Format("Version: {0}", value.Version)); sb.AppendLine(string.Format("CodingType: {0}", value.CodingType)); if (!string.IsNullOrEmpty(value.IBAN)) sb.AppendLine(string.Format("IBAN: {0}", value.IBAN)); if (!string.IsNullOrEmpty(value.CreditorAddressType)) sb.AppendLine(string.Format("Creditor address type: {0}", value.CreditorAddressType)); if (!string.IsNullOrEmpty(value.CreditorName)) sb.AppendLine(string.Format("Creditor name: {0}", value.CreditorName)); if (!string.IsNullOrEmpty(value.CreditorStreetOrAddressLine1)) sb.AppendLine(string.Format("Creditor street or address line 1: {0}", value.CreditorStreetOrAddressLine1)); if (!string.IsNullOrEmpty(value.CreditorBuildingNumberOrAddressLine2)) sb.AppendLine(string.Format("Creditor building number or address line 2: {0}", value.CreditorBuildingNumberOrAddressLine2)); if (!string.IsNullOrEmpty(value.CreditorTown)) sb.AppendLine(string.Format("Creditor town: {0}", value.CreditorTown)); if (!string.IsNullOrEmpty(value.CreditorCountry)) sb.AppendLine(string.Format("Creditor country: {0}", value.CreditorCountry)); if (!string.IsNullOrEmpty(value.Amount)) sb.AppendLine(string.Format("Amount: {0}", value.Amount)); if (!string.IsNullOrEmpty(value.AmountCurrency)) sb.AppendLine(string.Format("Amount currency: {0}", value.AmountCurrency)); if (!string.IsNullOrEmpty(value.UltimateDebtorAddressType)) sb.AppendLine(string.Format("Ultimate debtor address type: {0}", value.UltimateDebtorAddressType)); if (!string.IsNullOrEmpty(value.UltimateDebtorName)) sb.AppendLine(string.Format("Ultimate debtor name: {0}", value.UltimateDebtorName)); if (!string.IsNullOrEmpty(value.UltimateDebtorStreetOrAddressLine1)) sb.AppendLine(string.Format("Ultimate debtor street or address line 1: {0}", value.UltimateDebtorStreetOrAddressLine1)); if (!string.IsNullOrEmpty(value.UltimateDebtorBuildingNumberOrAddressLine2)) sb.AppendLine(string.Format("Ultimate debtor building number or address line 2: {0}", value.UltimateDebtorBuildingNumberOrAddressLine2)); if (!string.IsNullOrEmpty(value.UltimateDebtorTown)) sb.AppendLine(string.Format("Ultimate debtor town: {0}", value.UltimateDebtorTown)); if (!string.IsNullOrEmpty(value.UltimateDebtorCountry)) sb.AppendLine(string.Format("Ultimate debtor country: {0}", value.UltimateDebtorCountry)); if (!string.IsNullOrEmpty(value.PaymentReferenceType)) sb.AppendLine(string.Format("Payment reference type: {0}", value.PaymentReferenceType)); if (!string.IsNullOrEmpty(value.PaymentReference)) sb.AppendLine(string.Format("Payment reference: {0}", value.PaymentReference)); if (!string.IsNullOrEmpty(value.UnstructuredMessage)) sb.AppendLine(string.Format("Unstructured message: {0}", value.UnstructuredMessage)); if (!string.IsNullOrEmpty(value.BillInformation)) sb.AppendLine(string.Format("Bill information: {0}", value.BillInformation)); if (!string.IsNullOrEmpty(value.AlternativeSchemeParameters1)) sb.AppendLine(string.Format("Alternative scheme parameters 1: {0}", value.AlternativeSchemeParameters1)); if (!string.IsNullOrEmpty(value.AlternativeSchemeParameters1)) sb.AppendLine(string.Format("Alternative scheme parameters 2: {0}", value.AlternativeSchemeParameters2)); System.Console.WriteLine(sb.ToString()); } } /// <summary> /// Generates the Swiss QR Code barcode image. /// </summary> /// <param name="barcodeValue">The barcode value.</param> private static Vintasoft.Imaging.VintasoftBitmap Generate(Vintasoft.Barcode.BarcodeInfo.SwissQRCodeValueItem barcodeValue) { // create barcode writer using (Vintasoft.Barcode.BarcodeWriter writer = new Vintasoft.Barcode.BarcodeWriter()) { // encode Swiss QR Code barcode to the writer settings Vintasoft.Barcode.SymbologySubsets.BarcodeSymbologySubsets.SwissQRCode.Encode(barcodeValue, writer.Settings); // generate barcode image return writer.GetBarcodeAsVintasoftBitmap(); } } /// <summary> /// Recognizes the Swiss QR Code barcode from image. /// </summary> /// <param name="barcodeImage">The barcode image.</param> private static Vintasoft.Barcode.BarcodeInfo.SwissQRCodeBarcodeInfo Recognize(Vintasoft.Imaging.VintasoftBitmap barcodeImage) { // create barcode reader using (Vintasoft.Barcode.BarcodeReader reader = new Vintasoft.Barcode.BarcodeReader()) { // specify that Swiss QR Code barcode must be recognized reader.Settings.ScanBarcodeSubsets.Add(Vintasoft.Barcode.SymbologySubsets.BarcodeSymbologySubsets.SwissQRCode); // recognize barcodes in image Vintasoft.Barcode.IBarcodeInfo info = reader.ReadBarcodes(barcodeImage)[0]; // return information about recognized Swiss QR Code barcode return (Vintasoft.Barcode.BarcodeInfo.SwissQRCodeBarcodeInfo)info; } } }
System.Object
 Vintasoft.Barcode.BarcodeSymbology
   Vintasoft.Barcode.SymbologySubsets.BarcodeSymbologySubset
     Vintasoft.Barcode.SymbologySubsets.SwissQRCodeBarcodeSymbology
Целевые платформы: .NET 8; .NET 7; .NET 6; .NET Framework 4.8, 4.7, 4.6, 4.5, 4.0, 3.5
Члены типа SwissQRCodeBarcodeSymbology
Пространство имен Vintasoft.Barcode.SymbologySubsets